Route
The Route object
A route is essentially an ordered collection of destinations with route data describing the path, time and distance to get from one destination to the next. The route data is specified on the "to" destination, so the first (start) destination in a route always has routeData == null.
Destinations that make up a route can be destinations in jobs (determined by the jobId attribute), or they can be standalone destinations.
A route must consist of at least two destinations (start and end). The start and end destinations cannot have jobs associated with them.
Routes can also have resources scheduled on them but, unlike jobs, a route can only have one resource scheduled.
Attributes
This table does not include attributes that are considered trivial or self-explanatory. See also common attributes.
Attribute | Description |
---|---|
description | A free-text description of the route. May contain directions to the driver that applies to the entire route. |
destinations | The list of destinations |
startTime, endTime | Timestamp of route start/end. These are calculated from destination arrivalTimes. |
resource | Resources scheduled or assigned on this route. Currently only one allowed. |
state | State of the route. States are the same as job states. |
totalDistance | Total driving distance in meters of the entire route. |
totalTime | Total route time (excluding stops) in seconds of the entire route. |
totalStopDuration | Total duration of all stops in minutes. |
Example
The following route consists of 6 destinations (including start and end, 3 destinations have jobs). It is scheduled to a resource.
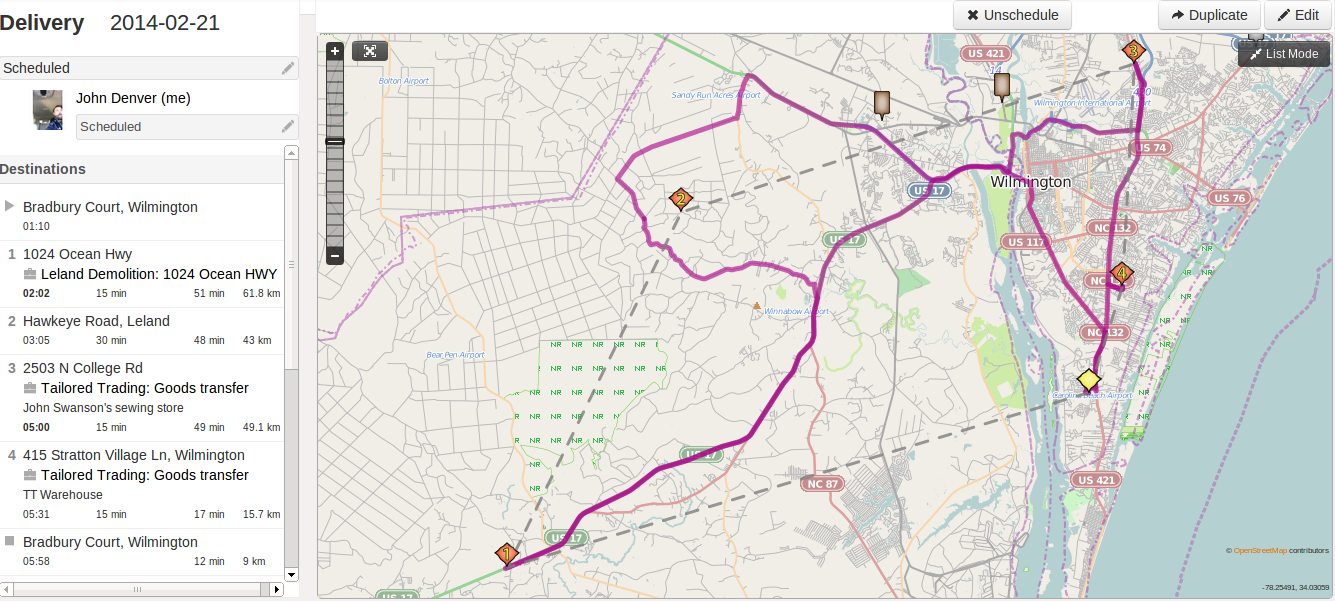
The route in the screenshot above would look like this in its JSON representation:
List Item Representation
Lists of routes do not include the following attributes:
- event
- destinations.routeData
List routes
GET
Paths
/route, /route/list/all | All routes | ||
/route/list/unassigned | Unassigned routes | ||
/route/list/mine | Routes scheduled to the token user |
Query Parameters
from | List routes from this date/time | Timestamp | 2013-01-01 |
to | List up to this date/time | Timestamp | 2013-01-05 |
states | Comma-separated list of states to include, except if fetching mine, then it is a list of resource states. Default: all states but DRAFT, FINISHED, CANCELED, CLOSED. | List | IN_TRANSIT,ON_STATION,STARTED |
untimed | Include routes that have no start time | Flag | |
current | Include unfinished routes even though their start time is before from | Flag | |
limit | Limit the number of returned objects | Integer | |
offset | Skip the first N objects | Integer | |
modified | Include only objects modified since this date/timestamp | Timestamp | 2015-10-05 |
Search routes
Search routes. Please note that the search representation contains only a subset of the detail and list representations.
Query Parameters
q |
Search query. Required.
The default search behavior is an ElasticSearch query, each search term ORed, with some fields boosted.
|
Example
GET /route/search?q=usa Content-type: application/json
- Method
- GET
- URL
- /route/search
- Authentication
- Token
- Response
- RouteSearchEntry object
Get a route
Create a route
Update or create a route
Update an existing route or create it (if it doesn't exist and :id is a UUID).
Example
PUT /route/0847ed17-b060-4599-a38c-be9a8b9cbb34
{ "title": "Delivery", "description": "An updated route", "destinations": [ /* ... */ ] }
Response
The updated route object.
- Method
- PUT
- URL
- /route/:id
- Authentication
- Token
- Request
- Route object
- Response
- Route object
Delete a route
Delete a route.
Example
DELETE /route/0847ed17-b060-4599-a38c-be9a8b9cbb34
- Method
- DELETE
- URL
- /route/:id
- Authentication
- Token
- Response
- Status code only
Schedule a user on a route
Schedule a user as a resource on a route.
Query Parameters
userId | ID or UUID of user, or me | ID | me |
sendMessage | Send a message to the user | Flag |
Example
PUT /route/0847ed17-b060-4599-a38c-be9a8b9cbb34/schedule?userId=dd738c3a-5a66-4f1c-bc03-52d2f4c03913&sendMessage
Response
The updated route object.
- Method
- PUT
- URL
- /route/:id/schedule
- Authentication
- Token
- Response
- Route object
Unschedule a user from a route
Unschedule a user as a resource from a route.
Query Parameters
userId | ID or UUID of user, or me | ID | me |
sendMessage | Send a message to the user | Flag |
Example
PUT /route/0847ed17-b060-4599-a38c-be9a8b9cbb34/unschedule?userId=dd738c3a-5a66-4f1c-bc03-52d2f4c03913&sendMessage
Response
The updated route object.
- Method
- PUT
- URL
- /route/:id/unschedule
- Authentication
- Token
- Response
- Route object
Routing Services
This API is unofficial
The resources described below are not part of our open API. They are made available to select customers/partners only. Please do not spread this documentation to third parties without consent from Coredination.
The following services act as a common interface to a number of third-party routing service providers. Coredination's service layer has a configurable way of determining which backend provider to select depending on the request. It also has caching, so if you make identical requests several times, it returns a cached response and doesn't have to bother the third party service provider.
Please note the URL path /routing as opposed to /route used for the information resource.
Even though the data format is the same, the routing services (with the /routing path) do not save the route. Typically, on the client side, you create the Route object, use the routing services described below to geocode, optimize and calculate the route, and then use POST /route or PUT /route/:uuid to save it.
Geocode destinations in a route
Geocoding means translating an address to a latitude/longitude location.
This service is essentially a broker of different third-party geocoding services on the backend, with a common data format.
Example
POST /routing/geocode
{ "destinations": [ { "location": { "placeName": "365 Golden Knights Blvd, FL" } } ] }
Response
The geocoded route object with the added (transient) places attribute containing a list of some more details on the geocoded result, one per each destination.
{ "destinations": [ { "arrivalTime": null, "calculatedArrivalTime": null, "id": null, "jobId": null, "jobTitle": null, "location": { "accuracy": null, "altitude": null, "bearing": null, "latitude": 30.49165, "longitude": -84.481639, "placeName": "365 Golden Knights Blvd, FL", "provider": "UNKNOWN", "speed": null, "timeStamp": 1392992008836 }, "locationDescription": null, "routeData": null, "routeDistance": null, "routeId": null, "routeTime": null, "stopDuration": null, "workItems": null } ], "places": [ { "accuracy": null, "area": null, "city": "Midway", "country": "United States", "countryCode": "US", "county": "Gadsden County", "distance": null, "latitude": 30.49165, "longitude": -84.481639, "name": "365 Knights Rd, Midway, FL 32343-2789, United States", "placeName": null, "population": null, "source": "Yahoo Public", "streetName": "Knights Rd", "streetNumber": 365, "type": "STREET_ADDRESS" } ], /* ... plus a bunch of other attributes */ }
- Method
- POST
- URL
- /routing/geocode
- Authentication
- Key
- Request
- Route object
- Response
- Route object
Optimize a route
Optimizing means reordering its destinations so that the cost is as low as possible. The meaning of cost is dependent on the algorithm.
The current implementation is a fairly basic Traveling Salesman Problem solver based on a genetic algorithm, where cost is simply the straight-line distance between destinations.
Note: A route has to be geocoded before it can be optimized.
Example
POST /routing/optimize
{ "destinations": [ /* ... */ ] }
Response
The route object with (possibly) reordered destinations.
- Method
- POST
- URL
- /routing/optimize
- Authentication
- Key
- Request
- Route object
- Response
- Route object
Calculate time/distance between destinations
This service is essentially a broker of different third-party routing services on the backend, with a common data format.
This calculates and sets the time and distance between destinations, and also the total for the entire route.
Note: A route has to be geocoded before it can be calculated (routed).
Example
POST /routing/route
{ "destinations": [ /* ... */ ] }
Response
The route object with times and distances filled in.
- Method
- POST
- URL
- /routing/route
- Authentication
- Key
- Request
- Route object
- Response
- Route object